Structual design patterns – What is the bridge design pattern simplified?
Bridge is part of Structural Design Patterns that decouples an abstraction from its implementation, allowing both to vary independently. What is the bridge design pattern used for? It is used to decouple the interface (the datatype) from its implementation, allowing them to be developed independently of one another, increasing flexibility and maintainability in complex software systems.
How does the Bridge Design Pattern work?
Let us get back to our old friend, the furniture factory (Design Patterns Articles). What we want now is to create different types of furniture (chairs and tables) using different materials (wood and metal). One possible approach can be:
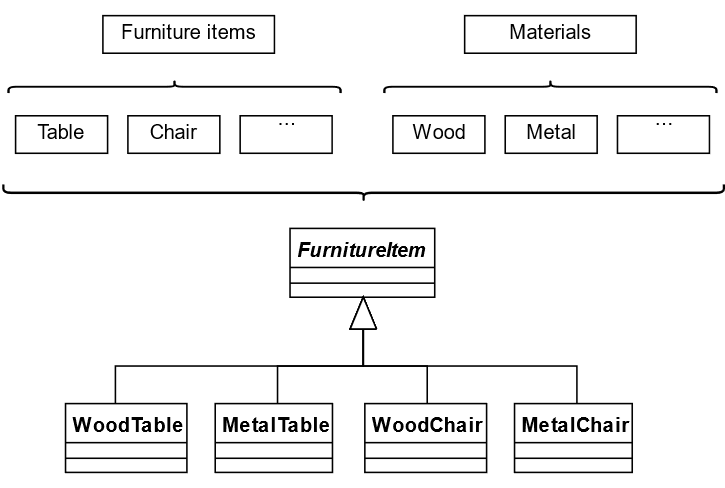
The number of class combinations grows in geometric progression
Adding new kinds of furniture items and materials to the hierarchy will grow it exponentially. For example, to add a bedside, we would need to introduce two subclasses, one for each material. And after that, adding a new material would require creating three subclasses, one for each type of furniture item. The further we go, the worse it becomes, a very common issue with class inheritance.
The Bridge pattern can be used to separate the furniture item types from the materials, allowing us to easily create new combinations without changing existing code. It helps solve the problem by switching from inheritance to object composition.
Following this approach, we can extract the material-related code into its own class with two subclasses: Wood and Metal. The FurnitureItem class then gets a reference field pointing to one of the material objects. Now the FurnitureItem can delegate any material-related work to the linked material object. That reference will act as a bridge between the FurnitureItem and Material classes. From now on, adding new materials will not require changing the furniture items hierarchy, and vice versa.
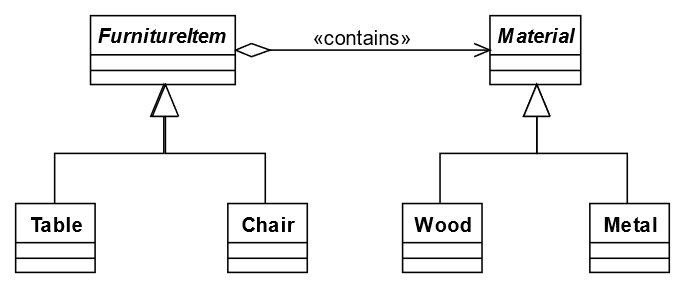
We can prevent the explosion of a class hierarchy by
transforming it into several related hierarchies
If we generalize the concepts presented till now, we will end up with the following UML diagram:
The Abstraction provides high-level control logic. It relies on an Implementation based object to do the actual low-level work. In our example, the Abstraction is materialized by the FurnitureItem abstract class. The Implementation declares the interface that is common to all concrete implementations.
An Abstraction derived object can only communicate with an Implementation based object via methods that are declared here. Although the Abstraction could utilize the same methods as the Implementation, it usually has some complex behaviors that rely on more than one of the operations declared by the Implementation.
In our example, the Implementation is materialized by the Material interface and the Concrete Implementation by the actual materials used for the furniture manufacturing: wood, metal, and so on.
Refined Abstractions provide variants of control logic. They, like their parents, work with various implementations via the general Implementation interface.
The Client is usually only interested in working with the Abstraction. However, it is the client’s responsibility to connect the Abstraction derived object to one of the Implementation based objects.
The source code for the presented sample is available on GitHub.
Advantages of the Bridge Design Pattern
- Decoupling Abstraction from Implementation: The primary advantage of the Bridge pattern is that it decouples the Abstraction and the Implementation, allowing them to evolve independently. This separation makes it easier to modify or extend both the Abstraction and the Implementation without affecting each other.
- Flexibility and Extensibility: The pattern provides a high level of flexibility by allowing us to combine different Abstractions with different Implementations at runtime. This makes it easy to add new variations without altering the existing code (Open/Closed Principle)
- Improved Maintainability: Since changes to the abstraction or implementation do not directly impact each other, maintenance becomes easier. We can modify one part without affecting the other, reducing the risk of unintended side effects.
- Reduced Complexity: In situations where we have multiple hierarchies or complex class structures, the Bridge pattern can help simplify the design by breaking down the problem into smaller, manageable parts.
- Enhanced Testing: With the Bridge pattern, we can create separate test cases for abstractions and implementations, enabling more focused and targeted testing.
- Parallel Development: Teams can work in parallel on the abstraction and implementation, speeding up the development process and improving collaboration.
Disadvantages of the Bridge Design Pattern
While the Bridge Design Pattern offers many benefits, it also comes with some potential disadvantages that should be considered when deciding whether to use it:
- Increased Complexity: While the pattern simplifies certain aspects of the design, it can also introduce additional complexity by requiring the creation of multiple classes and interfaces.
- Overhead: The introduction of additional layers and abstractions can sometimes lead to a small overhead in terms of runtime performance and memory usage.
- Learning Curve: Developers who are not familiar with the pattern might find it initially confusing due to the separation of concerns and the need to work with multiple classes and interfaces.
- Design Overhead: Applying the Bridge pattern might be overkill for simpler systems or cases where the abstraction and implementation are unlikely to change.
- Potential Over-Abstraction: In some cases, designers might overuse the pattern, creating unnecessary layers of abstraction that complicate the system without providing tangible benefits.
- Increased Code Volume: Implementing the Bridge pattern requires more code and classes, which can lead to a larger codebase. While this can improve maintainability, it might also make the code harder to understand for newcomers.
It is important to carefully consider these disadvantages in relation to our specific use case before deciding to employ the Bridge Design Pattern.
When should we use the Bridge Design Pattern?
The Bridge Design Pattern should be considered when we encounter certain scenarios or requirements in our software design. It is especially useful in situations where we need to manage complex hierarchies, anticipate changes in both Abstraction and Implementation, and maintain a separation between these two aspects. Here are some situations where we should consider using the Bridge pattern:
- Abstraction and Implementation Independence: When we want to design systems in a way that changes in the Abstraction (interface or core functionality) should not affect the Implementation (how that functionality is achieved) and vice versa.
- Multiple Hierarchies: When we have a system that has multiple hierarchies that intersect, and we want to avoid the combinatorial explosion of classes that might arise if we use traditional inheritance.
- Variability in Abstractions and Implementations: When we expect different variations or flavors of both the Abstraction and the Implementation. The Bridge pattern allows us to mix and match these variations easily.
- Platform-Dependent Code: When dealing with platform-specific code, such as code that interacts with different operating systems or hardware. The Bridge pattern can help manage these differences by encapsulating platform-specific code within implementations.
- Maintainability and Flexibility: When we want to create a design that is easier to maintain and extend. Changes to abstractions or implementations will not ripple through the entire codebase, reducing the risk of introducing bugs or unintended side effects.
- Third-Party Integrations: When integrating with third-party libraries or frameworks that might evolve or change. The Bridge pattern can provide a way to insulate our code from such changes.
- Testing and Debugging: When we want to improve testing and debugging by isolating the components we are testing from their implementations. This can make it easier to pinpoint issues.
- Parallel Development: When we are working with teams that can work on the abstraction and implementation independently. The Bridge pattern allows parallel development without tight coupling.
- Scalability: When we are building a system that needs to scale and evolve over time. The Bridge pattern’s separation of concerns can make it easier to handle changes and growth.
What is strategy vs bridge design pattern?
The Strategy Pattern and the Bridge Pattern are both structural design patterns, but they serve different purposes and address different design concerns. Let’s compare them:
- Strategy Pattern: The Strategy Pattern is a behavioral pattern that focuses on defining a family of algorithms, encapsulating each one as a separate class, and making them interchangeable. It allows a client to choose an algorithm from a family of algorithms at runtime.
- Bridge Pattern: The Bridge Pattern is a structural pattern that separates the abstraction from its implementation. It is used to decouple abstraction and implementation, allowing them to vary independently.
Real-world examples of Bridge Model Design Pattern
The Bridge Design Pattern is applied in various real-world software systems where a clear separation between Abstraction and Implementation is required. Here are a few examples:
- Graphics Libraries: Graphics libraries often use the Bridge pattern to separate the drawing primitives (Abstraction) from the specific rendering engines (Implementation). This allows the library to support multiple platforms or devices without changing the drawing code.
- Device Drivers: Operating systems use the Bridge pattern to manage device drivers. The Abstraction represents the generic device operations (e.g., read, write), while the Implementations are the similar solution structures for specific drivers in different hardware devices.
- GUI Frameworks: Graphical user interface frameworks employ the Bridge pattern to separate UI controls (Abstraction) from their platform-specific implementations (Implementation). This allows the same UI control to look and behave differently on different platforms.
- Database Systems: In database management systems, the Bridge pattern can be seen in the way database interfaces (Abstraction) are separated from the actual database engines (Implementation). Different databases can be supported by changing the Implementation while keeping the Abstraction intact.
- Notification Systems: Notification systems that deliver messages through different channels (email, SMS, push notifications) use the Bridge pattern. The Abstraction represents the notification message, while Implementations handle the delivery mechanisms.
- Vehicle Manufacturing Systems: In the context of vehicle manufacturing, the Bridge pattern can be used to separate the types of vehicles (Abstraction) from their propulsion systems (Implementation), allowing for a variety of vehicle types with different engines.
- Healthcare Information Systems: Healthcare systems that need to support multiple data storage solutions (e.g., relational databases, NoSQL databases) can benefit from the Bridge pattern to abstract the data storage operations.
These examples demonstrate how the Bridge pattern can be applied to various domains, providing flexibility, extensibility, and maintainability in software systems that need to accommodate multiple variations and interactions.
Conclusion
In conclusion, the Bridge Design Pattern can be highly beneficial when we anticipate changes, need to manage complexity, want to maintain a separation between Abstractions and Implementations, and desire a flexible and extensible design. It is especially valuable in scenarios where we need to manage various variations and interactions within our software system. However, it is important to consider the trade-offs and evaluate whether the benefits of flexibility and maintainability outweigh the potential complexity and overhead introduced by the pattern.